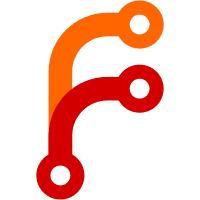
* Make `YamlLoader` generic on the type of the `Node`. This is required because deeper node need to have annotations too. * Add a `LoadableYamlNode` trait, required for YAML node types to be loaded by `YamlLoader`. It contains methods required by `YamlLoader` during loading. * Implement `LoadableYamlNode` for `Yaml`. * Take `load_from_str` out of `YamlLoader` for parsing non-annotated nodes. This avoids every user to specify the generics in `YamlLoader::<Yaml>::load_from_str`.
21 lines
644 B
Rust
21 lines
644 B
Rust
#[macro_use]
|
|
extern crate quickcheck;
|
|
|
|
use quickcheck::TestResult;
|
|
|
|
use saphyr::{load_from_str, Yaml, YamlEmitter};
|
|
|
|
quickcheck! {
|
|
fn test_check_weird_keys(xs: Vec<String>) -> TestResult {
|
|
let mut out_str = String::new();
|
|
let input = Yaml::Array(xs.into_iter().map(Yaml::String).collect());
|
|
{
|
|
let mut emitter = YamlEmitter::new(&mut out_str);
|
|
emitter.dump(&input).unwrap();
|
|
}
|
|
match load_from_str(&out_str) {
|
|
Ok(output) => TestResult::from_bool(output.len() == 1 && input == output[0]),
|
|
Err(err) => TestResult::error(err.to_string()),
|
|
}
|
|
}
|
|
}
|